NOTE: This website and documentation is under construction, so please check back later.
We are almost done publishing the JS GUI module and it’s getting way better that we first anticipated. We are very exited to release it soon. Please come back soon for the the release date.
– 2026-02-14
swing-ui
is an awesome graphical user interface module for Node.js that works on Windows, Linux and Mac. All you need is Node.js and Java 11+ (JRE or JDK) on your system to be able to create beautiful interactive window forms, buttons, text editor, lists, tables, progress bars, split panels, menu, submenu, context menu, tray icon, toolbar, file chooser, color picker, dialog windows, check box, radio box, sliders and much more.
This module takes Jeff Atwood‘s famous remark about JavaScript to the next level:
“Any application that can be written in JavaScript, will eventually be written in JavaScript”
– Jeff Atwood
You can track version updates of swing-ui module at https://www.npmjs.com/package/swing-ui where I publish new releases.
I will be publishing video tutorials on my YouTube Channel how to use this GUI module to create beautiful desktop applications and all of its components, so see you there.
Demo Application
Here is a small example of such application written purely in JavaScript. I made it to stay organized with all data that I need to remember and work with.
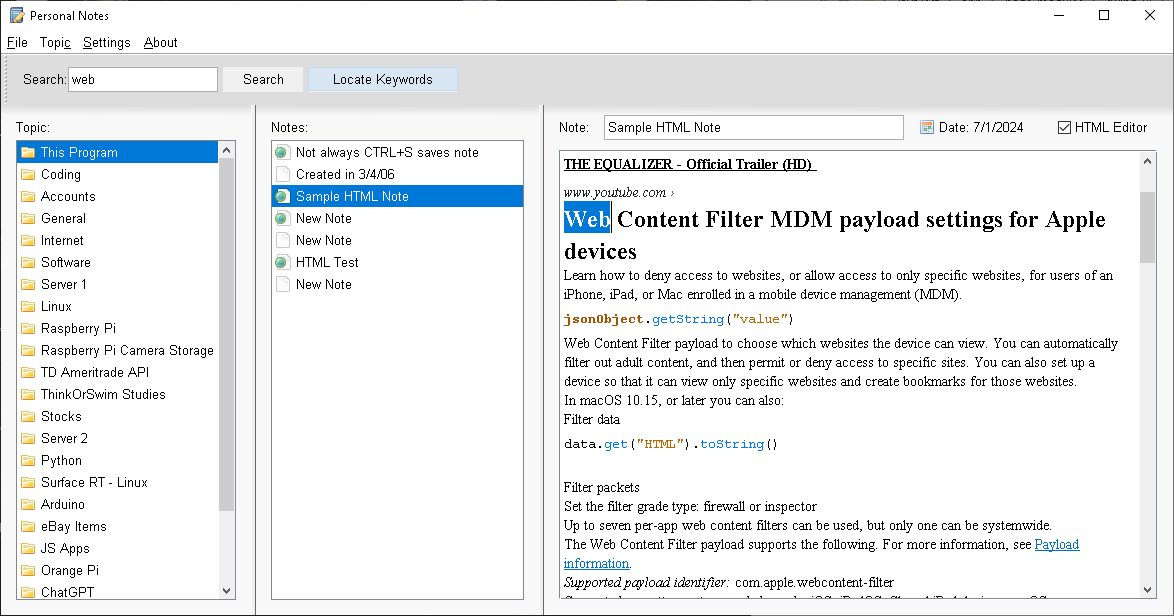
Demo
Here is a simple demonstration of syntax used to build a desktop application using swing-ui module.
require("swing-ui");
MyApp = new class extends Window
{
constructor()
{
super();
this.text("My Application").closeOperation(Window.CloseOperation.Exit).size(600, 400).location(200, 200).visible(true);
this.textfield1 = new TextArea().addTo(this).size(555, 60).location(15, 15).text("Hello World\r\nThis is a DEMO application.");
this.button1 = new Button("Clear Text").addTo(this).size(100, 40).location(15, 90).onAction(function(e){MyApp.textfield1.text("You pressed button: " + this.text());});
this.button2 = new Button("Close").addTo(this).size(100, 40).location(125, 90).text("Close").onAction(e=>{this.close();});
}
};
This example generates the following application with functional buttons.
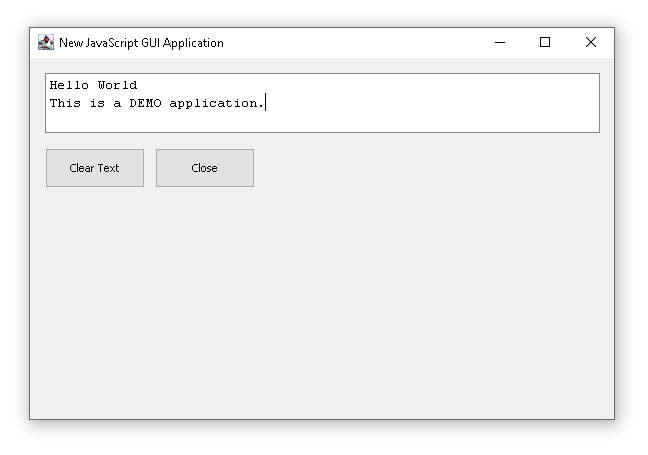
Coding Style
I tried hard to make it as easy as possible to use, so even beginners should be able to use it with no problem. So long as they reference the documentation, they shouldn’t have much struggle getting things done. As you can see in demo code above, most notable feature of coding with swing-ui is its fluent interface using method chaining. Using it makes lines longer than usual, but it allows for much less lines than using equal sign = to set UI component properties.
Get Started
Check out the installation page to see step by step how to start building your first application in few minutes, or even seconds.